1. question: 将有序数组转换为二叉搜索树(简单)
给你一个整数数组 nums ,其中元素已经按 升序 排列,请你将其转换为一棵 高度平衡 二叉搜索树。
高度平衡 二叉树是一棵满足「每个节点的左右两个子树的高度差的绝对值不超过 1 」的二叉树。
来源:力扣(LeetCode)
链接:https://leetcode.cn/problems/convert-sorted-array-to-binary-search-tree
示例 1:
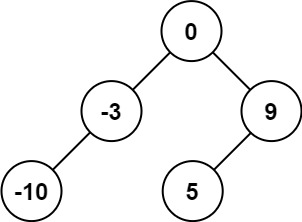
1 2 3
| 输入:nums = [-10,-3,0,5,9] 输出:[0,-3,9,-10,null,5] 解释:[0,-10,5,null,-3,null,9] 也将被视为正确答案:
|
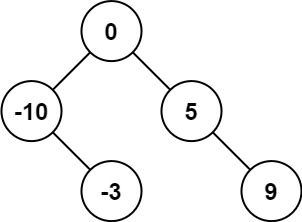
示例 2:
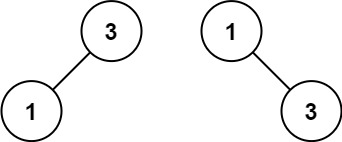
1 2 3
| 输入:nums = [1,3] 输出:[3,1] 解释:[1,null,3] 和 [3,1] 都是高度平衡二叉搜索树。
|
提示:
1 2 3
| 1 <= nums.length <= 104 -104 <= nums[i] <= 104 nums 按 严格递增 顺序排列
|
2. answers
这道题没什么可说的。构造二叉搜索树,只需要右边大于根节点大于左节点即可。构造高度平衡的树,只需要选取根节点的时候,选择有序数组的中间值即可。代码如下所示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59
| public class Solution_0076 {
public TreeNode recurConstruct(int[] nums, int start, int end) {
if(start == end) return new TreeNode(nums[start]);
int mid = (start + end) / 2;
TreeNode root = new TreeNode(nums[mid]);
if(mid > start) root.left = recurConstruct(nums, start, mid - 1);
if(mid < end) root.right = recurConstruct(nums, mid + 1, end);
return root; }
public TreeNode sortedArrayToBST(int[] nums) {
return recurConstruct(nums, 0, nums.length - 1); }
public static void main(String[] args) { System.out.println();
int[] nums = {1, 3};
Solution_0076 s = new Solution_0076();
TreeNode result = s.sortedArrayToBST(nums);
Queue<TreeNode> queue = new LinkedList<>();
queue.offer(result);
while(queue.size() > 0 ) {
int length = queue.size();
while(length-- > 0) {
result = queue.poll();
System.out.print(result.val + "\t");
if(result.left != null) queue.offer(result.left); if(result.right != null) queue.offer(result.right);
}
System.out.println(); }
} }
|
3. 备注
参考力扣(LeetCode)官网 - 全球极客挚爱的技术成长平台 (leetcode-cn.com),代码随想录 (programmercarl.com)。