1. question: 路径总和 II(中等)
给你二叉树的根节点 root 和一个整数目标和 targetSum ,找出所有 从根节点到叶子节点 路径总和等于给定目标和的路径。
叶子节点 是指没有子节点的节点。
来源:力扣(LeetCode)
链接:https://leetcode.cn/problems/path-sum-ii
示例 1:
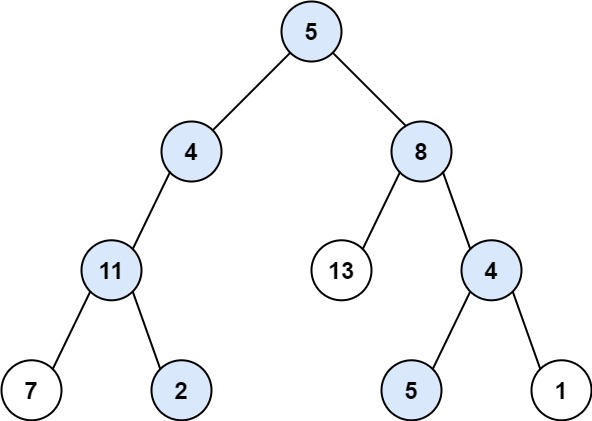
1 2
| 输入:root = [5,4,8,11,null,13,4,7,2,null,null,5,1], targetSum = 22 输出:[[5,4,11,2],[5,8,4,5]]
|
示例 2:
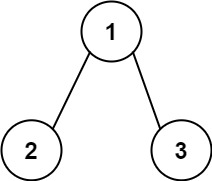
1 2
| 输入:root = [1,2,3], targetSum = 5 输出:[]
|
示例 3:
1 2
| 输入:root = [1,2], targetSum = 0 输出:[]
|
提示:
1 2 3
| 树中节点总数在范围 [0, 5000] 内 -1000 <= Node.val <= 1000 -1000 <= targetSum <= 1000
|
2. answers
这道题和前面那道题类似,区别在于,这道题需要找出所有的路径,并且输出的是路径。其实找所有路径不难,难点在于怎么保存路径。因为以递归的思路来看,只有到达叶子节点时,才能知道到底能否满足要求,但是高层的节点已经遍历完了,我们不知道应不应该保存。
实际上,递归是会逐层返回高层的,完全可以在递归的时候返回当前层满足条件的子路径。逐层扩充到高层即可。代码如下所示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102
| public class Solution_0062 {
public static List<List<Integer>> getPath(TreeNode tn, Integer targetSum) {
List<List<Integer>> result = new ArrayList<>();
if(tn.left == null && tn.right == null) { if(targetSum - tn.val == 0) {
List<Integer> temp = new ArrayList<>(); temp.add(tn.val); result.add(temp); }
return result; }
List<List<Integer>> left = new ArrayList<>(); List<List<Integer>> right = new ArrayList<>();
if(tn.left != null) left = getPath(tn.left, targetSum - tn.val);
if(tn.right != null) right = getPath(tn.right, targetSum - tn.val);
for(List<Integer> list: left) {
List<Integer> temp = new ArrayList<>();
temp.add(tn.val); temp.addAll(list);
result.add(temp); }
for(List<Integer> list: right) {
List<Integer> temp = new ArrayList<>();
temp.add(tn.val); temp.addAll(list);
result.add(temp); }
return result; }
public static List<List<Integer>> pathSum(TreeNode root, int targetSum) {
List<List<Integer>> result = new ArrayList<>();
if(root == null) return result;
result = getPath(root, targetSum);
return result;
}
public static void main(String[] args) {
List<List<Integer>> result = pathSum(null, 4);
for(List<Integer> list: result) { for(Integer i: list) System.out.print(i + "\t"); System.out.println(); } } }
|
3. 备注
参考力扣(LeetCode)官网 - 全球极客挚爱的技术成长平台 (leetcode-cn.com),代码随想录 (programmercarl.com)。