1. question: 反转链表(简单)
给你单链表的头节点 head ,请你反转链表,并返回反转后的链表。
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/problems/reverse-linked-list
示例 1:
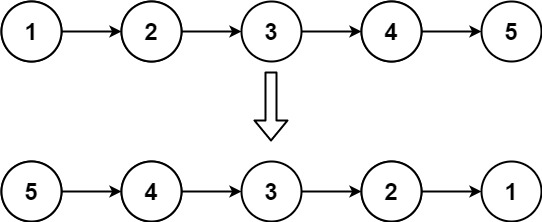
1 2
| 输入:head = [1,2,3,4,5] 输出:[5,4,3,2,1]
|
示例 2:
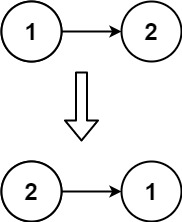
1 2
| 输入:head = [1,2] 输出:[2,1]
|
示例 3:
提示:
1 2
| 链表中节点的数目范围是 [0, 5000] -5000 <= Node.val <= 5000
|
2. answers
这道题没什么可说的,就是遍历链表,依次将节点插入到新链表的头节点和链表中间。代码如下所示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| public class Solution_0036 {
public static ListNode reverseList(ListNode head) {
ListNode newHead = new ListNode(); ListNode temp;
while(head != null) {
temp = head.next;
head.next = newHead.next; newHead.next = head;
head = temp; }
return newHead.next; }
public static void main(String[] args) {
ListNode ln1 = new ListNode(5); ListNode ln2 = new ListNode(4, ln1); ListNode ln3 = new ListNode(3, ln2); ListNode ln4 = new ListNode(2, ln3); ListNode ln5 = new ListNode(1, ln4);
System.out.println();
ListNode result = reverseList(ln5);
while(result != null) { System.out.println(result.val);
result = result.next; } } }
public class ListNode { int val; ListNode next; ListNode() {} ListNode(int val) { this.val = val; } ListNode(int val, ListNode next) { this.val = val; this.next = next; } }
|
3. 备注
参考力扣(LeetCode)官网 - 全球极客挚爱的技术成长平台 (leetcode-cn.com),代码随想录 (programmercarl.com)。