1. question: 平衡二叉树(简单)
给定一个二叉树,判断它是否是高度平衡的二叉树。
本题中,一棵高度平衡二叉树定义为:
一个二叉树每个节点 的左右两个子树的高度差的绝对值不超过 1 。
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/problems/balanced-binary-tree
示例 1:
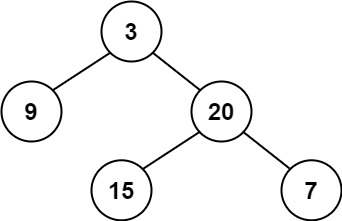
1 2
| 输入:root = [3,9,20,null,null,15,7] 输出:true
|
示例 2:
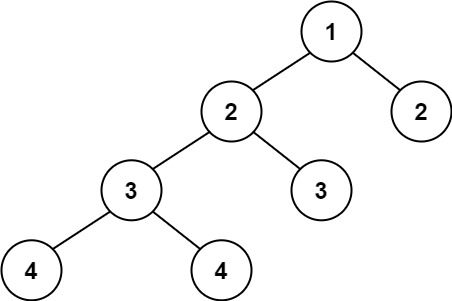
1 2
| 输入:root = [1,2,2,3,3,null,null,4,4] 输出:false
|
示例 3:
提示:
1 2
| 树中的节点数在范围 [0, 5000] 内 -104 <= Node.val <= 104
|
2. answers
注意,这里将高度和深度混为一谈,均为高度的意思。
这道题最直观的方法就是求解左右子树的高度,然后判断高度差。注意,如果高度差相差小于等于1并不意味着一定是平衡二叉树,因为可能左右子树根本就不是平衡二叉树。所以还需要判断左右子树是否是平衡二叉树。
代码如下所示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69
| public class Solution_0025 {
public static boolean isBalanced(TreeNode root) {
if(root == null) { return true; }
int left_depth = getDepth(root.left); int right_depth = getDepth(root.right);
if(Math.abs(left_depth - right_depth) > 1) { return false; }
boolean leftResult = isBalanced(root.left); boolean rightResult = isBalanced(root.right);
return leftResult && rightResult; }
public static int getDepth(TreeNode root) {
if(root == null) { return 0; }
Queue<TreeNode> queue = new LinkedList<>(); queue.offer(root); int length, depth = 0;
while(!queue.isEmpty()) {
length = queue.size(); depth ++; while(length > 0) { root = queue.poll();
if(root.left != null) { queue.offer(root.left); }
if(root.right != null) { queue.offer(root.right); }
length --;
} }
return depth; }
public static void main(String[] args) { System.out.println();
TreeNode tn1 = new TreeNode(15); TreeNode tn2 = new TreeNode(7); TreeNode tn3 = new TreeNode(20, tn1, tn2); TreeNode tn4 = new TreeNode(9); TreeNode tn5 = new TreeNode(3, tn3, tn4);
System.out.println(isBalanced(tn5)); } }
|
仔细分析一下,其实上述层序遍历求解高度的操作重复了很多次(每次递归都要层序遍历一次,而且每次递归都重复遍历了)。这其实是没必要的。怎么优化呢?
在求解深度的时候,其实就要判断该节点是否为平衡二叉树。
怎么判断呢?左右孩子节点的高度差不大于1.(如果是,那么该节点的高度就是孩子节点高度的最大值加1;如果不是,就直接返回-1,无需后续的判断了)
因此,这就形成了递归。
代码如下所示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| public class Solution_0025_02 {
public static boolean isBalanced(TreeNode root) {
return getDepth(root) != -1; }
public static int getDepth(TreeNode root) {
if(root == null) { return 0; }
int left = getDepth(root.left);
if(left == -1) { return -1; }
int right = getDepth(root.right);
if(right == -1) { return -1; }
if(Math.abs(left - right) > 1) { return -1; }
return Math.max(left, right) + 1; }
public static void main(String[] args) {
TreeNode tn1 = new TreeNode(15); TreeNode tn2 = new TreeNode(7); TreeNode tn3 = new TreeNode(20, tn1, tn2); TreeNode tn4 = new TreeNode(9); TreeNode tn5 = new TreeNode(3, tn3, tn4);
System.out.println(isBalanced(tn5)); } }
|
3. 备注
参考力扣(LeetCode)官网 - 全球极客挚爱的技术成长平台 (leetcode-cn.com),代码随想录 (programmercarl.com)。