1. question: 二叉树的层序遍历(中等)
给你二叉树的根节点 root ,返回其节点值的 层序遍历 。 (即逐层地,从左到右访问所有节点)。
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/problems/binary-tree-level-order-traversal
示例 1:
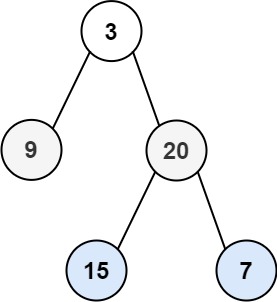
示例1
| 输入:root = [3,9,20,null,null,15,7] 输出:[[3],[9,20],[15,7]]
|
示例 2:
示例 3:
提示:
| 树中节点数目在范围 [0, 2000] 内 -1000 <= Node.val <= 1000
|
2. answers
思路:
层序遍历比较简单,直接用队列存储节点,将节点的左右孩子节点按顺序存入队列即可。然后再取出即可。
关键的一个问题是,如何将一层的节点封装成一个List。即怎么判断从队列中取出的节点是否属于当前层。
一种思路是:在遍历的时候,记录其下一层孩子节点的数量。当从队列中取元素的时候,判断已取出的数量是否达到了该层的数量,如果达到了,则将已有的List加入到结果中,并新建空的List。
代码如下所示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93
| public class Solution_0013 {
public static List<List<Integer>> levelOrder(TreeNode root) {
List<List<Integer>> result = new ArrayList<>(); List<Integer> subResult = new ArrayList<>();
if(root == null) { return result; }
Queue<TreeNode> queue = new LinkedList<>(); queue.offer(root);
Queue<Integer> nodeChilds = new LinkedList<>();
nodeChilds.offer(1);
int offerLength = 0;
int pollLength = nodeChilds.poll();
while(!queue.isEmpty()) {
if(pollLength == 0) { result.add(subResult); subResult = new ArrayList<>();
pollLength = nodeChilds.poll(); }
root = queue.poll();
subResult.add(root.val); pollLength -= 1;
if(root.left != null) { queue.offer(root.left); offerLength += 1; }
if(root.right != null) { queue.offer(root.right); offerLength += 1; }
if(offerLength != 0 && pollLength == 0) {
nodeChilds.offer(offerLength); offerLength = 0; } }
result.add(subResult);
return result; }
public static void main(String[] args) {
TreeNode tn1 = new TreeNode(7); TreeNode tn2 = new TreeNode(15); TreeNode tn3 = new TreeNode(20, tn2, tn1);
TreeNode tn4 = new TreeNode(9);
TreeNode root = new TreeNode(3, tn4, tn3);
List<List<Integer>> result = levelOrder(root); for(List<Integer> i: result) { for(Integer j : i) { System.out.print(j + " "); } System.out.println(); } } }
|
思路2:
上面的思路其实没问题,但是实现过程变复杂了。保存下一层要遍历的节点数量,只需要用一个变量代替即可,不需要用队列。而且下一层要遍历的节点数量,实际上就是循环开始时队列的长度。
代码如下所示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64
| public class Solution_0013_03 {
public static List<List<Integer>> levelOrder(TreeNode root) {
List<List<Integer>> result = new ArrayList<>();
if(root == null) { return result; }
Queue<TreeNode> queue = new LinkedList<>();
queue.offer(root);
int length = 1;
while(!queue.isEmpty()) {
length = queue.size(); List<Integer> subResult = new ArrayList<>();
while(length > 0) {
root = queue.poll();
subResult.add(root.val);
if(root.left != null) { queue.offer(root.left); }
if(root.right != null) { queue.offer(root.right); }
length --; }
result.add(subResult); }
return result; }
public static void main(String[] args) { TreeNode tn1 = new TreeNode(7); TreeNode tn2 = new TreeNode(15); TreeNode tn3 = new TreeNode(20, tn2, tn1);
TreeNode tn4 = new TreeNode(9);
TreeNode root = new TreeNode(3, tn4, tn3);
List<List<Integer>> result = levelOrder(root); for(List<Integer> i: result) { for(Integer j : i) { System.out.print(j + " "); } System.out.println(); } } }
|
3. 备注
参考力扣(LeetCode)官网 - 全球极客挚爱的技术成长平台 (leetcode-cn.com),代码随想录 (programmercarl.com)。