1. question: 二叉树的后续遍历(简单)
给你一棵二叉树的根节点 root ,返回其节点值的 后序遍历 。
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/problems/binary-tree-postorder-traversal
示例 1:
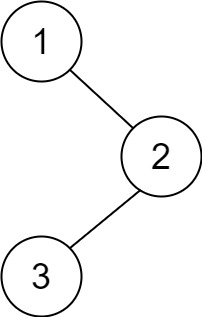
1 2
| 输入:root = [1,null,2,3] 输出:[3,2,1]
|
示例 2:
示例 3:
提示:
1 2
| 树中节点的数目在范围 [0, 100] 内 -100 <= Node.val <= 100
|
2. answers
和前面一样,二叉树的后序遍历也分为递归和迭代两种方法。
2.1 递归解法
代码如下所示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| public class Solution_0012 {
public static void recurive(TreeNode root, List<Integer> result){
if(root != null) { recurive(root.left, result); recurive(root.right, result); result.add(root.val); } }
public static List<Integer> postorderTraversal(TreeNode root) {
List<Integer> result = new ArrayList<>();
recurive(root, result);
return result; }
public static void main(String[] args) { TreeNode tn1 = new TreeNode(3); TreeNode tn2 = new TreeNode(2, tn1, null); TreeNode root = new TreeNode(1, null, tn2);
List<Integer> integers = postorderTraversal(root);
for(Integer i: integers){ System.out.println(i); } } }
|
2.2 迭代解法
注意,后续遍历的迭代解法和前序遍历“类似”。在前序遍历中,左右子树访问顺序修改一下,即中右左,然后再将结果反转一下,就是后续遍历左右中了。
代码如下所示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57
| public class Solution_0012 {
public static List<Integer> postorderTraversalPlus(TreeNode root) {
List<Integer> result = new ArrayList<>();
Stack<TreeNode> sta = new Stack<>();
if( root != null) { sta.push(root); }
while(!sta.isEmpty()) { root = sta.pop();
result.add(root.val);
if(root.left != null) { sta.push(root.left); }
if(root.right != null) { sta.push(root.right); } }
Collections.reverse(result);
return result; }
public static void main(String[] args) { TreeNode tn1 = new TreeNode(3); TreeNode tn2 = new TreeNode(2, tn1, null); TreeNode root = new TreeNode(1, null, tn2);
List<Integer> integers = postorderTraversalPlus(root); for(Integer i: integers){ System.out.println(i); } } }
|
3. 备注
参考力扣(LeetCode)官网 - 全球极客挚爱的技术成长平台 (leetcode-cn.com),代码随想录 (programmercarl.com)。